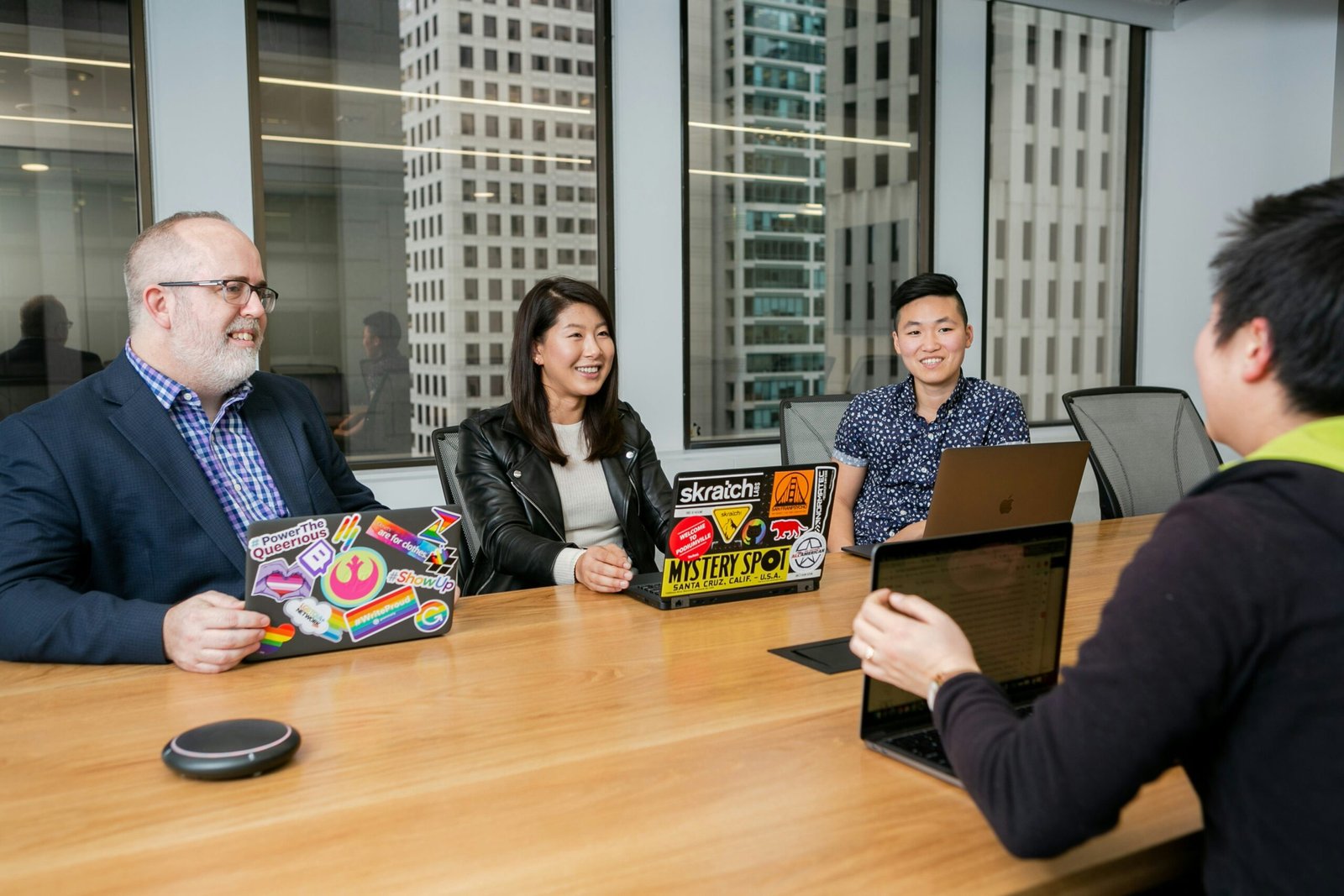
Introduction to APIs in Mobile Development
Application Programming Interfaces (APIs) serve as vital components in the architecture of mobile app development. They act as intermediaries that allow different software applications to communicate, enabling a seamless exchange and interaction of data. In the realm of mobile development, the integration of APIs can greatly enhance the functionality and responsiveness of apps, providing users with a richer experience by connecting them to various services and data sources.
APIs present an organized way for developers to access specific functionalities of an application or service without needing to understand its internal workings. This abstraction not only streamlines the development process but also promotes the ability to build on existing systems. For instance, through REST or SOAP protocols, a mobile app can retrieve user data from a cloud server, send it back to the server for processing, or even integrate third-party services such as payment gateways, mapping tools, or social media platforms. Such interactions significantly enrich mobile applications, making them more versatile and capable of meeting user needs.
Furthermore, the significance of APIs extends beyond mere data exchange. They also serve as a means of implementing security measures and managing user authentication through tokens or API keys, which ensures that data integrity is maintained throughout the communication process. Developers can take advantage of these secure connections to build robust applications that prioritize user privacy and data protection.
In summary, APIs are an indispensable aspect of mobile app development. By leveraging APIs, developers are able to create applications that not only function smoothly but also provide access to a wide array of features and services. This connectivity ultimately leads to vibrant, user-friendly applications that can adapt to evolving demands and integrate seamlessly into users’ daily lives.
Types of APIs Used in Mobile Apps
Application Programming Interfaces (APIs) play a crucial role in mobile app development, facilitating interaction between different software components. Among the numerous types of APIs utilized in this arena, RESTful APIs, SOAP APIs, and GraphQL are some of the most prominent. Each type offers unique characteristics and advantages, making them suitable for various mobile app scenarios.
RESTful APIs are based on the Representational State Transfer architectural style and use standard HTTP methods such as GET, POST, PUT, and DELETE. Known for their simplicity and efficiency, RESTful APIs allow mobile app developers to retrieve and manipulate data seamlessly. They are stateless, allowing for greater scalability and responsiveness, which is particularly beneficial for apps that require frequent updates or interactions with remote servers. Their widespread adoption stems from their compatibility with diverse platforms, making them a popular choice for developing mobile applications.
On the other hand, SOAP APIs (Simple Object Access Protocol) adhere to a protocol for exchanging structured information in web services. While SOAP APIs tend to be more complex than RESTful APIs, they offer robust security features, making them suitable for applications that necessitate high levels of security and reliability. Businesses that handle sensitive data often opt for SOAP APIs to adhere to stringent compliance standards.
GraphQL represents a more modern advancement in API design, allowing clients to request only the data they need. This flexibility enhances performance by minimizing the amount of data transferred between the server and client. GraphQL’s ability to aggregate data from multiple sources into a single query streamlines the development of mobile apps focusing on improving user experience and reducing load times.
In conclusion, understanding the diverse types of APIs is essential for mobile app developers seeking to choose the most appropriate solution for their projects. By leveraging the unique benefits of RESTful APIs, SOAP APIs, and GraphQL, developers can create more efficient, secure, and user-friendly applications. Each API type caters to specific needs, ensuring that mobile applications can effectively meet user demands while maintaining optimal performance.
Understanding API Documentation
API documentation serves as a crucial resource for developers looking to integrate application programming interfaces (APIs) into mobile applications. Well-structured documentation provides vital information that outlines how to effectively utilize an API. The importance of thorough API documentation cannot be understated, as it facilitates clarity and efficiency in the integration process.
Key components typically found in API documentation include endpoints, which represent specific paths through which API requests are made. Each endpoint corresponds to a unique function or resource, allowing developers to access data or invoke various services provided by the API. Additionally, the request methods, such as GET, POST, PUT, and DELETE, are crucial for determining how to interact with these endpoints effectively. Understanding the appropriate methods to use for each interaction is essential for successful implementation, and clear documentation helps streamline this process.
Another important aspect covered in API documentation is the authentication requirements necessary to access the API. Many APIs require a key or token to ensure that only authorized users can access specific features or data, making it essential for developers to familiarize themselves with these requirements prior to integration. Moreover, understanding response structures, which detail how data will be returned, allows for better handling of the received information and smoother error management.
Navigating API documentation can sometimes be overwhelming, but certain strategies can aid in comprehension. Developers should begin by familiarizing themselves with the table of contents to locate relevant sections quickly. Additionally, taking notes or creating reference guides while reviewing the documentation can enhance understanding and retention of key information. By recognizing these essential elements and utilizing effective navigation techniques, developers can significantly improve their ability to integrate APIs into mobile applications.
Setting Up Your Development Environment
Establishing a solid development environment is crucial for successful API integration in mobile app development. The tools, libraries, and frameworks you select will significantly influence the efficiency of your workflow and the quality of your application. For developers targeting Android, the primary tool is Android Studio, which offers an Integrated Development Environment (IDE) tailored to facilitate the development process. Android Studio comes equipped with powerful features such as code completion, debugging tools, and a built-in emulator for testing purposes.
In the context of iOS app development, Xcode is the equivalent IDE that developers must utilize. Xcode includes essential tools like Interface Builder for designing user interfaces and a powerful simulator for testing applications on different device types. Both Android Studio and Xcode allow developers to manage dependencies easily, often through package managers like Gradle for Android and CocoaPods or Swift Package Manager for iOS. These package managers enable the integration of APIs and libraries seamlessly, maintaining an organized project structure.
Furthermore, it is vital to integrate libraries that facilitate API calls and data handling. For Android, libraries such as Retrofit and Volley can be extremely helpful, providing a simple way to connect to RESTful APIs. In the iOS ecosystem, Alamofire serves a similar purpose by offering an elegant dependency for performing network requests. Additionally, developers should familiarize themselves with configuring environment variables and endpoints, ensuring a smooth connection to external services while maintaining security constraints.
By carefully selecting the right tools and frameworks, and implementing best practices for dependency management, developers can create a robust and efficient environment dedicated to fruitful API integration. This strategic setup not only enhances productivity but also lays the groundwork for future scalability as projects evolve.
Making API Calls: Step-by-Step Guide
Making API calls is a crucial aspect of mobile app development, allowing the application to interact with external services and retrieve or send data. The first step in this process is to set up the necessary dependencies and libraries that facilitate API interaction. Most mobile development frameworks provide built-in libraries or APIs to create network requests efficiently. For instance, in Android, you can use libraries such as Retrofit or Volley, while in iOS, URLSession is commonly employed.
Once the environment is prepared, you will need to determine the type of API call required, typically categorized as GET, POST, PUT, or DELETE. A GET request is used for retrieving data, while POST sends new data to the server. To initiate an API call, construct the URL endpoint according to the API documentation. Ensure that the request includes any required headers, such as content type or authentication tokens, to ensure successful communication with the API.
Next, make the API call using the selected library. For example, in Android using Retrofit, you would define an interface for your API calls and invoke the method corresponding to your request type. After executing the call, you will handle the response. A successful response usually provides the data in JSON or XML format, which you must parse appropriately to utilize the information in your app.
Error handling is vital when making API calls, as numerous issues can arise, including network timeouts, invalid responses, or server errors. Implementing try-catch blocks or callback functions ensures that any errors are captured and processed gracefully. Debugging techniques, such as logging responses and using tools like Postman for testing API endpoints, can help identify and troubleshoot common problems. Through this structured approach to API calls, developers can create more robust and reliable mobile applications.
Handling Data in Mobile Apps
In the context of mobile app development, handling data retrieved from Application Programming Interfaces (APIs) is an essential aspect that ensures seamless functionality and user experience. APIs often deliver data in structured formats such as JSON (JavaScript Object Notation) and XML (eXtensible Markup Language), which are both widely recognized for their versatility and ease of use. JSON is predominantly favored for its lightweight structure, making it particularly suitable for mobile applications, while XML may be utilized in scenarios where document storage is necessary.
Once the data is retrieved, the next step involves parsing, a process that transforms the received data into a format that the mobile application can easily manipulate. For JSON, numerous libraries and frameworks, such as GSON or Jackson for Android, provide straightforward methods for parsing, allowing developers to convert JSON strings into Java objects. Similar libraries exist for iOS, such as SwiftyJSON, which simplifies the handling of JSON data. On the other hand, XML parsing can be accomplished using tools like XMLPullParser for Android or the built-in XMLParser for iOS, which help in reading the data tree efficiently.
After parsing, effectively storing and displaying the data within user interfaces is paramount. Local storage solutions like SQLite or Room for Android and Core Data for iOS can be utilized to retain data on the device, enabling offline access and improved performance. Additionally, it is crucial to design user interfaces that present the data in a clear and intuitive manner. Utilizing frameworks such as React Native or SwiftUI can enhance user experience by providing modern and responsive UI components that elegantly display the API data.
Overall, by understanding the data formats, implementing parsing methods, and optimizing data management strategies, developers can create mobile applications that not only connect effectively with APIs but also provide users with access to rich, dynamic, and personalized content.
Authentication and Security Considerations
In mobile app development, integrating Application Programming Interfaces (APIs) necessitates a careful examination of authentication and security considerations to protect user data and ensure a secure user experience. One of the most widely adopted authentication methods in this context is OAuth, which allows applications to access user data without compromising security credentials. By utilizing OAuth, developers can implement delegated access, meaning users can authorize a third-party application to perform actions or retrieve data on their behalf without sharing their passwords directly. This method significantly improves security and enhances the user experience.
Another common approach is the use of API keys. API keys serve as a unique identifier for the client requesting access to the API. However, since these keys can be easily exposed, developers should incorporate stringent mechanisms to safeguard them. For instance, API keys should be stored securely in a back-end server rather than hardcoded into the mobile application, diminishing the risk of unauthorized access. Additionally, employing methods like IP whitelisting can restrict API calls to trusted sources, further bolstering security.
Best practices for securing API calls also involve the use of HTTPS whenever possible to encrypt data in transit. This ensures that any sensitive information exchanged between the mobile application and the server cannot be easily intercepted by malicious parties. It’s crucial to implement proper error handling and logging within the application as well, as these practices help in monitoring for unusual behavior and potential security breaches.
Overall, to create a safe environment for users, mobile app developers must prioritize and integrate robust authentication methods alongside stringent security protocols. By doing so, they protect both user data and the integrity of the application itself, fostering trust and loyalty within the user base.
Testing and Debugging APIs in Mobile Apps
Testing and debugging APIs in mobile app development is a crucial step that ensures seamless integration and optimal performance. Effective strategies can help developers identify issues early in the development process and improve the overall user experience. To achieve this, leveraging specialized tools such as Postman and Charles Proxy can be immensely beneficial.
Postman is widely used for testing APIs due to its user-friendly interface and robust feature set. Developers can use Postman to make API requests, analyze responses, and verify that the API behaves as expected. By sending different types of requests (GET, POST, PUT, DELETE), developers can validate the effectiveness and responsiveness of their API integrations under various conditions. Additionally, Postman allows for the automation of tests, which is essential for continuous integration/continuous deployment (CI/CD) practices.
Charles Proxy, another valuable tool, helps in debugging API calls made by mobile applications. By acting as an intermediary between the mobile app and the server, Charles Proxy allows developers to capture and inspect network traffic. This visibility facilitates the identification of issues such as misconfigured endpoints, inefficient response times, or unexpected error messages. Charles Proxy can also assist in simulating various network conditions, allowing for more comprehensive testing.
Unit testing is another vital component of testing APIs in mobile applications. Writing unit tests for the API integration logic ensures that individual components behave as expected in isolation. This practice not only makes the codebase more reliable but also simplifies future modifications, as regressions can be quickly identified and addressed. Common pitfalls to avoid during this phase include neglecting edge cases, overlooking error handling, and failing to account for asynchronous operations, all of which could lead to compromised functionality.
By employing the right tools and methodologies, developers can ensure that the API integrations within their mobile apps are robust and perform well, ultimately leading to a better experience for end users.
Real-world Examples and Case Studies
To illustrate the significance of APIs in mobile app development, several successful applications can serve as noteworthy case studies. One prominent example is the Uber application, which has effectively integrated multiple APIs to enhance its functionality. Through the Google Maps API, Uber provides users with real-time navigation and accurate location tracking, allowing for a seamless ride-hailing experience. By leveraging payment processing APIs such as Stripe and Braintree, the app simplifies the transaction process for users, thus enhancing customer satisfaction. However, challenges related to API failures and latency issues occasionally arose, which the developers addressed by implementing caching mechanisms to improve load times and ensure a smoother user experience.
Another pertinent example is the Spotify mobile application, which employs APIs to offer users access to an extensive music library. The Spotify Web API allows developers to retrieve information about songs, albums, and playlists, which facilitates personalized user experiences. The challenge of managing user data privacy is especially crucial in this context, and Spotify navigates it by adhering to strict data protection regulations while integrating its API. The implementation of OAuth, an authorization protocol, further ensures secure access to user accounts, allowing users to maintain control over their data while enjoying the app’s services.
Moreover, the Airbnb mobile application exemplifies effective API integration by utilizing a combination of payment APIs and location-based services. This enables users to search for accommodations while facilitating secure payment transactions. The primary challenge faced during this integration process included ensuring compatibility between various APIs, which was overcome through comprehensive testing and optimization strategies. Such meticulous attention to detail promotes a smooth user experience, showcasing the critical role of APIs in creating successful, functional mobile applications. These case studies exemplify how innovative API integration fosters robust app capabilities while addressing various development hurdles.